Leon Böttger’s GoogleFindMyTools is a re-implementation of Google’s Find My Device network. It works with Android devices and commercial trackers, but experimental support for ESP32-based Bluetooth trackers has recently been added.
The implementation features two components. First, the main.py Python script that will list and locate devices, and then the ESP32 firmware implemented in C with the ESP-IDF. The host computer will also need several Python libraries that can be installed with “pip install -r requirements.txt” and Google Chrome web browser.
This is the output of the Python script on my Ubuntu laptop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
jaufranc@CNX-LAPTOP-5:~/edev/sandbox/GoogleFindMyTools$ python main.py Loading... [UsernameProvider] Username was not setup yet. Type your Google Username (Email without @gmail.com)') and press 'Enter':[email protected] [FCMReceiver] Credentials updated. [FCMReceiver] Listening for notifications. [AuthFlow] This script will now open Google Chrome on your device to login to your Google account. > Please make sure that Chrome is installed on your system. > For macOS users only: Make that you allow Python (or PyCharm) to control Chrome if prompted. [AuthFlow] Press Enter to continue... [AuthFlow] Installing ChromeDriver... [AuthFlow] ChromeDriver installed and browser started. [AuthFlow] Waiting for 'oauth_token' cookie to be set... [AuthFlow] Retrieved Account Token successfully. The following trackers are available: 1. Oppo A98 5G: xxxx-0000-2551-xxxx-xxxxxxxx If you want to see locations of a tracker, type the number of the tracker and press 'Enter'. If you want to register a new ESP32-based tracker, type 'r' and press 'Enter': |
After logging in to my Google account, the script could locate my smartphone. From there, I can either get the smartphone’s GPS coordinate by selecting 1 or register an ESP32-based Bluetooth tracker. I don’t have any but let’s give it a try anyway:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
If you want to register a new ESP32-based tracker, type 'r' and press 'Enter': r Loading... [SharedKeyRetrieval] You need to log in again to access end-to-end encrypted keys to decrypt location reports. This script will now open Google Chrome on your device. Make that you allow Python (or PyCharm) to control Chrome (macOS only). [SharedKeyRetrieval] Press 'Enter' to continue... [SharedKeyFlow] Signed in successfully. [SharedKeyFlow] Received Shared Key. Registered device successfully. Copy the Advertisement Key below. It will not be shown again. Afterward, go to the folder 'GoogleFindMyTools/ESP32Firmware' and follow the instructions in the README.md file. +------------------------------------------------------------------------------+ | 2acbd5cd41b7acbedef192f0141cd5b4a62345df | | Advertisement Key | +------------------------------------------------------------------------------+ |
I had to log in to Google again for the script to retrieve a shared key, and I was given an advertisement key (that I modified for this post) that needs to be copied and pasted into line 15 of main.c replacing “INSERT_YOUR_ADVERTISEMENT_KEY_HERE”:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
#include <stdio.h> #include <stdint.h> #include <string.h> #include <stdlib.h> #include "esp_bt.h" #include "esp_gap_ble_api.h" #include "esp_log.h" #include "nvs_flash.h" // For NVS functions like nvs_flash_init #include "esp_bt_main.h" // For esp_bluedroid_* functions #include "esp_err.h" // For error handling #define TAG "ESP_FMDN" // This is the advertisement key / EID. Change it to your own EID. const char *eid_string = "INSERT_YOUR_ADVERTISEMENT_KEY_HERE"; // Function to convert a hex string into a byte array void hex_string_to_bytes(const char *hex, uint8_t *bytes, size_t len) { for (size_t i = 0; i < len; i++) { sscanf(hex + 2 * i, "%2hhx", &bytes[i]); } } |
This should be done in Visual Studio with the ESP-IDF framework installed, and from there you can build the firmware and flash it to an ESP32 tracker connected to the host over USB.
We can run main.py again and the phone and the newly registered ESP32 tracker can be found by the script:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
jaufranc@CNX-LAPTOP-5:~/edev/sandbox/GoogleFindMyTools$ python main.py Loading... The following trackers are available: 1. Oppo A98 5G: xxxx-0000-2551-xxxx-xxxxxxxx 2. GoogleFindMyTools ESP32: xxxx-0000-2ad2-xxxx-xxxxxxxx If you want to see locations of a tracker, type the number of the tracker and press 'Enter'. If you want to register a new ESP32-based tracker, type 'r' and press 'Enter': 1 [LocationRequest] Requesting location data for device with canonic ID: xxxx-0000-2551-xxxx-xxxxxxxx [FCMReceiver] Listening for notifications. [FCMReceiver] Notification received! [LocationRequest] Location request successful. ---------------------------------------- [DecryptLocations] Decrypted Locations: Latitude: 11.3347438 Longitude: 10.8444803 Altitude: 2580 Time: 2025-02-12 09:58:34 Status: 1 Is Own Report: True ---------------------------------------- |
Since I don’t have a tracker, I tried it with my phone, but the results should look similar with a working ESP32 tracker which relies on the phones in the Google Find My Device network for location. Don’t worry, I modified the value above for privacy…
It looks great but there are some known issues specific to the ESP32 implementation:
- Right now, you might have to re-register the ESP32 after 3 days, but Leon is working on a fix
- Users with new accounts should use Find My Device app on an Android device before registering a new ESP32 tracker (I didn’t need to do that).
- Locations for the ESP32 trackers can only be found via the Python script and not in the Google Find My Device app or website
- Privacy features such as rotating MAC addresses are not implemented
- The ESP32 firmware is optimized to find as many network reports as possible and not for battery life. You can change TX power and advertising interval parameters in main.c to lower the power consumption.
You’ll find the full instructions to give it a try yourself and the source code on GitHub. As a side note, if you are interested in open-source solutions for Trackers, you may want to check out the AirGuard project working with Android or iOS smartphones to locate Samsung Trackers (SmartTags), Google Find My Network Trackers, and Apple AirTags.
Via Hackaday
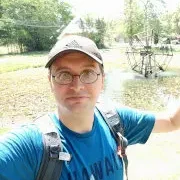
Jean-Luc started CNX Software in 2010 as a part-time endeavor, before quitting his job as a software engineering manager, and starting to write daily news, and reviews full time later in 2011.
Support CNX Software! Donate via cryptocurrencies, become a Patron on Patreon, or purchase goods on Amazon or Aliexpress